React and Angular Applications: React in Angular (Part 1)
We are the team working on the KKR Investor Portal. This is a web application, based on microservices. And we’re trying to follow this paradigm in all parts of the project.
We started from the microservice based backend, and this is a common approach, described in tons of books and articles on the Internet. But our frontend initially was a classic single page application written in Angular.
And we wanted to apply the microservices paradigm to the front end as well. The idea was to build new small web applications instead of extending an old one and connect them together via SSO and the user interface seamlessly.
Also, we wanted to migrate from Angular to React. Reasons, why we preferred React, are out of the scope of this post.
After months of development, we have three SPA instead of one:
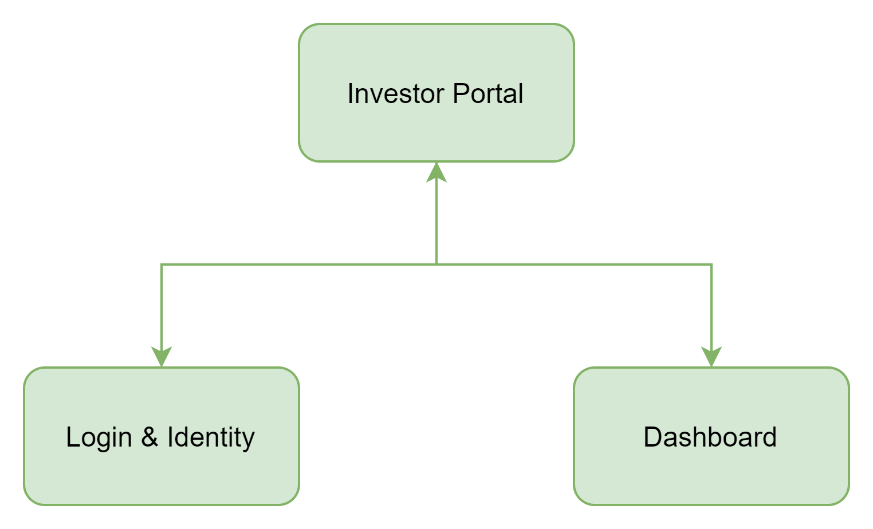
Investor Portal is written in Angular, and contains statements and documents, cashflows, and investor IDs.
Dashboard is written in React, and contains an investment summary, recent documents, capital calls, fee notices, etc.
Login & Identity app is written in React, which allows a user to change credentials and login options and navigate across applications.
All applications share a Single Sign-On authentication, so every application is aware of the user identity, and when a user is logged out from one application the user is logged out from the other two immediately.
From the user interface perspective, all three apps look and behave like a single application. A user just clicks Dashboard tab or Documents tab and gets into different applications based on different frameworks, but there’s no difference in front of his eyes.
Soon after we got to this point, we realized that there’s a significant part of the frontend functionality that’s duplicated across apps.
The most obvious parts were the header with all the navigation and the footer. Later the Feedback button was added to this. You must re-implement them in Angular and in React, but they are identical and do identical things. Every application should have its own version of the header which looks the same and do the same. If you want to change a style or add another tab, you should repeat this in all applications. And the number of applications grows.
What do developers do when they have functions re-usable in different applications? They create a library.
For web application, the common form of the library is a Node package (or npm package). It’s easy to include in any Angular or React application with the command:
npm install [package name]
And you can call any function or use any module from the package. Easily.
On the other hand, you don’t have to repeat the code in every application. You make your change in one place and all applications get your update after upgrading the package.
So, all we need is to move the header and the footer code into the npm package and reuse the package in all applications. Bingo!
Not actually.
You remember, we have two applications written in different frameworks, even in different languages. The Portal is Angular and Typescript language, whereas the Dashboard is React and JavaScript language. If you copy the code from React app and paste it to Angular app, it just won’t work. And vice versa. We need something else.
The idea of embedding Angular code to a React application doesn’t seem real. Angular is a framework, not a library, and Angular has its own HTML template engine, which makes its embedding to React application inhumanly difficult. It would be an unnecessary waste of time.
Thus, we can try to embed a React code into the Angular application. It’s possible because React is more a set of libraries than a framework.
Naturally, the first we should do is googling. Because most likely somebody already solved this problem.
Not actually. Not that simple.
There’s just one ready and working solution on the Internet – the Microsoft Angular-React (https://github.com/microsoft/angular-react).
And what are they saying about their solution:
“Both the core and fabric libraries are in production use in consumer-facing applications at Microsoft. That said, we (the team that currently maintains this project) are a product team, and @angular-react is not our primary focus. We maintain this because we need it and we share it with the wider community with the hope that it will prove useful to others. Of course, we attempt to provide help when possible and we love getting pull requests for improvements/enhancement/fixes from community members. But we don't have any specific plans for the future of this project.
Please take this into consideration when evaluating this project's suitability for your own needs.”
Doesn’t sound inspiring, isn’t it? We decided that the solution for what developers don’t have any specific plans for the future isn’t robust enough to base on.
But what do we have on our hands then? Nothing but experimenting.
The idea was to implement a React-Angular wrapper class that would be a classic Angular component that includes a React component, prepares all needed data for it and passes the data via React properties. This wrapper could be used in the Angular application because it’s the Angular component, but it could use the ReactDom.render for displaying a React component.
And after some time spent experimenting, we got this code:
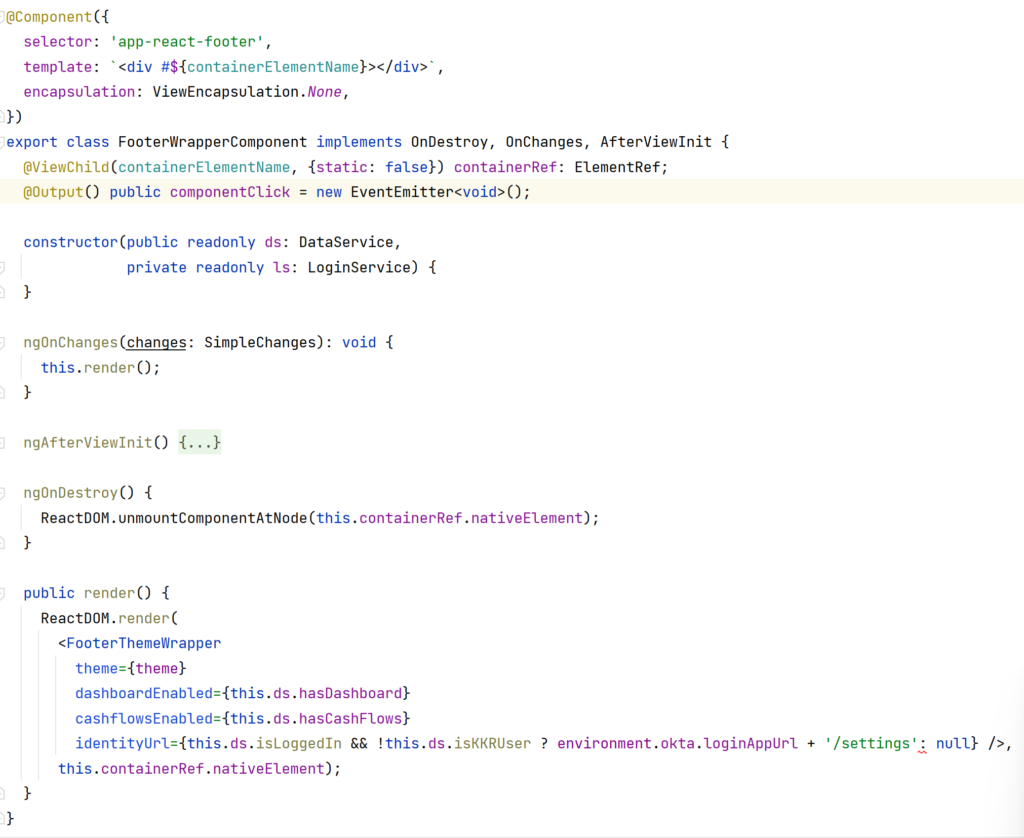
The FooterWrapperComponent is a classic Angular component.
The most important parts here are these three:
- the template that contain the placeholder #${containerElementName}
- @ViewChild(containerElementName, {static: false}) containerRef: ElementRef;
- ReactDOM.render that uses this.containerRef.nativeElement
This is the working chain that connects React and Angular. All the magic is here. Basically, we render the FooterThemeWrapper which is the React component in the place of the #${containerElementName} placeholder using ReactDOM.render.
And another duty of the FooterWrapperComponent is gathering all the data and callback functions (if needed) and passing the data to the properties of the FooterThemeWrapper. And we can see this in the code above.
Besides this, we have to include react and react-dom packages to package.json.
And add these options to tsconfig.json:
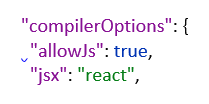
These options allow JavaScript code to be compiled in Angular and React to be used for .jsx React components we add to the app.
Disclaimer
The views expressed in each blog post are the personal views of each author and do not necessarily reflect the views of KKR. Neither KKR nor the author guarantees the accuracy, adequacy or completeness of information provided in each blog post. No representation or warranty, express or implied, is made or given by or on behalf of KKR, the author or any other person as to the accuracy and completeness or fairness of the information contained in any blog post and no responsibility or liability is accepted for any such information. Nothing contained in each blog post constitutes investment, legal, tax or other advice nor is it to be relied on in making an investment or other decision. Each blog post should not be viewed as a current or past recommendation or a solicitation of an offer to buy or sell any securities or to adopt any investment strategy. The blog posts may contain projections or other forward-looking statements, which are based on beliefs, assumptions and expectations that may change as a result of many possible events or factors. If a change occurs, actual results may vary materially from those expressed in the forward-looking statements. All forward-looking statements speak only as of the date such statements are made, and neither KKR nor each author assumes any duty to update such statements except as required by law.